Authentication is a crucial aspect of modern web applications, enabling developers to restrict access to certain features or data to authorized users only. In applications built with Node.js and Express, authentication is commonly implemented using tokens, with JSON Web Tokens (JWT) being the preferred choice due to their security and efficiency.
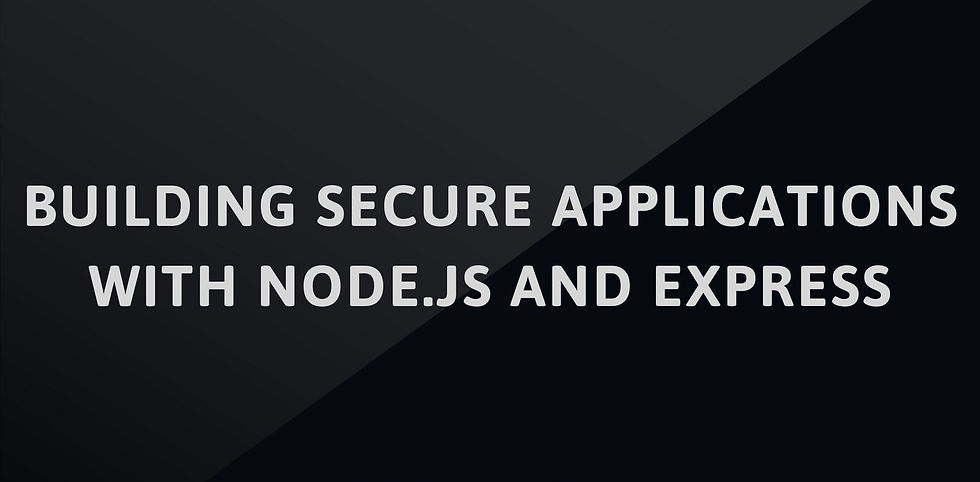
In this article, we'll explore how to set up user authentication in a Node.js and Express application using JWTs. By the end, you'll have a solid understanding of how to implement secure authentication for your own projects.
Understanding Authentication
Authentication refers to the process of confirming the identity of a user or system. In the context of web applications, this typically involves verifying user credentials such as a username and password. Once the verification is successful, the system grants the user access to the application. To bolster security, token-based authentication methods, like JSON Web Tokens (JWT), are widely adopted.
Why JSON Web Tokens (JWT)?
JSON Web Tokens (JWT) are recognized as an industry standard (RFC 7519) for secure and stateless token-based authentication. They enable secure information transmission between parties in the form of a JSON object. Tokens help verify a user's identity without the need to maintain session data on the server, making JWT an ideal choice for stateless applications.
Step-by-Step Guide: Implementing Authentication in Node.js and Express
Let’s walk through the steps to create a basic authentication system using JWT in a Node.js and Express application.
1. Setting Up Your Node.js Application
Before implementing authentication, you'll need to establish a basic Node.js and Express application. Use the following commands to initialize your project:
mkdir auth-demo
cd auth-demo
npm init -y
npm install express bcryptjs jsonwebtoken mongoose dotenv
Dependency Breakdown:
express: Framework for building the Node.js server.
bcryptjs: For securely hashing and comparing passwords.
jsonwebtoken: For creating and validating JWTs.
mongoose: To interact with MongoDB.
dotenv: For managing sensitive environment variables.
2. Configuring Environment Variables
Create a .env file in your project’s root directory to store sensitive data such as your database URI and JWT secret key:
MONGODB_URI=mongodb://localhost:27017/auth-demo
JWT_SECRET=your_jwt_secret_key
3. Connecting to MongoDB
In the root of your project, create a db.js file within a config folder to manage the MongoDB connection:
// config/db.js
const mongoose = require('mongoose');
const dotenv = require('dotenv');
dotenv.config();
const connectDB = async () => {
try {
await mongoose.connect(process.env.MONGODB_URI, {
useNewUrlParser: true,
useUnifiedTopology: true,
});
console.log('MongoDB connected');
} catch (err) {
console.error('Error connecting to MongoDB:', err.message);
process.exit(1);
}
};
module.exports = connectDB;
4. Creating the User Model
Next, define a User model to outline the structure of user documents in MongoDB. Inside a models folder, create User.js:
// models/User.js
const mongoose = require('mongoose');
const userSchema = new mongoose.Schema({
username: { type: String, required: true, unique: true },
password: { type: String, required: true },
});
module.exports = mongoose.model('User', userSchema);
5. Implementing User Registration
Now, let's establish a route for user registration. Create authController.js inside a controllers folder and implement the registration logic:
// controllers/authController.js
const User = require('../models/User');
const bcrypt = require('bcryptjs');
const jwt = require('jsonwebtoken');
// User registration
exports.register = async (req, res) => {
const { username, password } = req.body;
try {
const existingUser = await User.findOne({ username });
if (existingUser) {
return res.status(400).json({ message: 'Username already exists' });
}
const hashedPassword = await bcrypt.hash(password, 10);
const newUser = new User({ username, password: hashedPassword });
await newUser.save();
res.status(201).json({ message: 'User registered successfully' });
} catch (err) {
res.status(500).json({ error: err.message });
}
};
This function hashes the password with bcrypt before saving the user’s details in MongoDB.
6. Implementing User Login
Login is essential for generating and returning JWTs that clients will use for authenticating future requests. Here’s how to implement the login functionality:
// controllers/authController.js (continued)
exports.login = async (req, res) => {
const { username, password } = req.body;
try {
const user = await User.findOne({ username });
if (!user) {
return res.status(401).json({ message: 'Invalid username or password' });
}
const isPasswordValid = await bcrypt.compare(password, user.password);
if (!isPasswordValid) {
return res.status(401).json({ message: 'Invalid username or password' });
}
const token = jwt.sign({ id: user._id }, process.env.JWT_SECRET, { expiresIn: '1h' });
res.json({ token });
} catch (err) {
res.status(500).json({ error: err.message });
}
};
Upon a successful login, this function generates a JWT using jsonwebtoken and sends it back to the client.
7. Setting Up Middleware for Protected Routes
JWTs can be used to secure routes requiring authentication. Create middleware to verify tokens, ensuring that only authorized users can access certain endpoints:
// middleware/authMiddleware.js
const jwt = require('jsonwebtoken');
exports.verifyToken = (req, res, next) => {
const token = req.headers['authorization'];
if (!token) return res.sendStatus(403);
jwt.verify(token, process.env.JWT_SECRET, (err, user) => {
if (err) return res.sendStatus(403);
req.user = user;
next();
});
};
8. Applying Authentication Middleware
Finally, apply the middleware to protect a route. For instance, you might want users to access their profile only after logging in:
// routes/userRoutes.js
const express = require('express');
const { verifyToken } = require('../middleware/authMiddleware');
const { getUserProfile } = require('../controllers/userController');
const router = express.Router();
router.get('/profile', verifyToken, getUserProfile);
module.exports = router;
The verifyToken middleware checks for a valid JWT in the request headers, allowing access to the route if the token is valid.
Conclusion
In this article, we explored the key elements of implementing user authentication with JWT in a Node.js and Express application. We detailed the processes of user registration, login, and securing routes with token-based authentication. With this foundation, you can develop robust and secure authentication systems in your applications.
As you progress, consider adding features like refresh tokens, password reset options, and multi-factor authentication to enhance security. By mastering authentication with Node.js and Express, you’re on the path to creating scalable and secure web applications.
Comments